How I stopped failing coding interviews: a 7-step formula
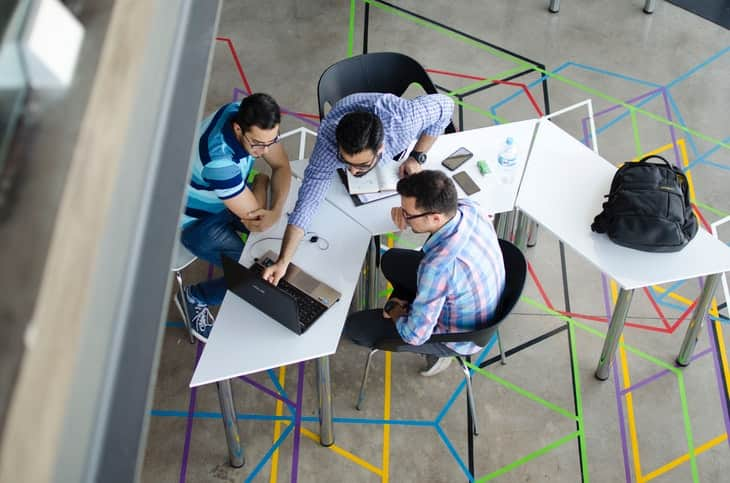
Failed a coding interview? Hey, me too! Technical interviews are hard. I've failed more than I can count.
But with time and practice, I realized there's a formula you can use to greatly improve your chances of successโeven if you don't get to the perfect solution. (And did you know that in some cases, you can get the right solution, but still fail overall if you approach the interview wrong? Yep, really.)
It took me several years, a lot of rejections, and becoming an interviewer myself to figure out that companies are looking for more than just the correct answer. Studying and understanding the right topics is important, but how you approach the interview is often what actually makes the difference.
My process for answering technical interview questions can be broken down into 7 steps. I'm going to share it here in case it helps anyone else playing that stressful game of finding a new role.
Why are technical interviews so hard?
A coding interview is a type of interview where you are given a puzzle, problem or exercise and asked to solve it by writing code. It's common for these interviews to test your knowledge of computer science, as they often require the use of a particular data structure and/or algorithm in order to reach an elegant solution.
So not only do you have to be able to reason about a problem, design a solution, and translate that solution into codeโyou have to do all this on the spot, under pressure, while your interviewer watches and assesses your performance.
We can argue about whether or not this is a good way to assess a candidate's skills (spoiler: it mostly isn't), but the reality is that interviews are not going away. And while some companies are better at designing their interview processes than others, we might as well go in prepared to deal with all eventualities.
My formula for passing coding interviews
Most technical interviews go like this: brief introduction from your interviewer, possibly a chance to introduce yourself, and then you're given technical problem to solve. They want to give you as much time to spend on the problem as possible.
At this point, many people (my past self included) jump right in and start writing code. This is the first mistake.
Here's what you should do instead:
- Write down sample input/output to confirm your understanding
- Ask questions about types, error handling and exceptional cases
- Come up with test cases
- Discuss, plan and write pseudocode
- Write the actual code
- Step through line by line with test cases
- Optimize and discuss additional considerations
1. Write down sample input/output to confirm your understanding
No matter how obvious it might seem, it's always worth checking you've actually understood the question properly.
Plenty of people fail interviews by answering the wrong question. You could code a perfect working solution, but if it's a solution to a different problem than the one you were asked to solve, you're probably not going to pass.
For example, take these two questions:
Write a program that prints the first n numbers in the Fibonacci sequence.
Write a program that prints all the numbers in the Fibonacci sequence up to n.
It would be easy enough to mistake one for the other, especially under pressure when you just want to get going with the interview.
Let's assume the interviewer had asked you the first of those two questions. To confirm your understanding, you could say something like:
To check my understanding, I'm assuming n will be an integer?
So, given an example input of n=3, the expected output would be 0, 1, 1
.
Is that correct?
Personally, I make a point of actually writing down these sample inputs/outputs. It makes it extra clear that you and the interviewer are on the same page, and can also be useful to reference in a later part of the interview. More on that later.
Taking a minute to do this simple check before you do anything else has two benefits:
- It stops you wasting time by going down the wrong path and having to be corrected, or worse, failing the entire interview by answering the wrong question
- It shows the interviewer you care about attention to detail, and can follow instructions accurately (both traits you definitely want to demonstrate)
2. Ask questions about types, error handling and exceptional cases
So, you've confirmed you get the basic idea of what the question is asking. Now it's time to dig a little deeper into your assumptions.
Companies love to ask questions with intentional ambiguity. They want you to ask clarifying questions. It's part of the test to see if you can spot when you don't have all the necessary information to solve a problem: do you ask the right questions to seek out the missing informationโor do you just make some assumptions and start coding anyway?
This is where you ask clarifying questions on things like input/output types, how you should handle errors, or any exceptional cases that could occur.
Here are some examples:
- Will the input always be an number, or should I be prepared to handle different types, e.g.
"3"
instead of3
? - Could the input be a negative number, or zero? Always an integer or could it be a floating point? Is there any upper/lower limit?
- How would you like me to handle other types of incorrect inputs (
null
,array
, etc.)? I could throw an error, or use a default value? - Should the output be an array of integers? Should it be formatted any particular way?
Chances are your interviewer won't care too much about some of these things, and will leave it up to you to choose how you handle them. But the point of asking these questions is to show that you sweat the small stuff, and take the time to think things through in detail.
3. Come up with test cases
Using the knowledge you gained from the answers in the previous step, you should now have a clear picture of what kind of inputs your program needs to handle.
You should think about the standard case of input/output (you can use the example you wrote down in step 1), but then also come up with a similar test case for each non-standard input or edge case.
Think about all the cases you just asked the interviewer about, and how your program should handle all those inputs.
Taking the Fibonacci example again, some test cases to consider could be:
Input: 3
Output: [0, 1, 1]
Input: 0
Output: []
Input: -3
Output: Error ('Input must be zero or greater')
Input: 10000000
Output: Error ('Input must be 1000000 or smaller')
Input: null
Output: Error ('Input must be an integer')
If you have time and are working in a real coding environment, it's a good idea to write these as actual unit tests that you can run. Not many people do this, so it's a great way to stand out and demonstrate that you know how to write good tests as well as good code.
But if not (e.g. you're writing on a whiteboard), be sure to at least write down the inputs and expected outputs for each case so you can manually check against them later.
4. Discuss, plan and write pseudocode
Now you're crystal clear on what your program needs to do, it's time to plan how it's going to do it. But, crucially, you're still not going to write actual code in this step.
This is the step where you think out loud, discussing the different approaches you could take, and their pros and cons (there will always be more than one way you could solve an interview problem).
Always start by mentioning the naive or brute force solution. The idea is not to actually end up coding this as your answer, but to use it as a starting point for discussion, so you can talk about how other solutions would be better (plus, you can always use it as a fallback option if you struggle to come up with anything more elegant).
As you're discussion solutions, this is the time to mention time/space complexity in terms of big O notation. This isn't necessary for every question or at every company, but it is certainly expected in FAANG-style interviews. Either way, it doesn't hurt to show you're able to reason about problems in those terms.
Let's imagine a different example. Say you're given a list of numbers. Your task is to write a program to determine if one particular number is in the list. The list is sorted in ascending order.
You could start the discussion like this:
Well, a brute force approach would be to start at the beginning and walk through the array, checking each number along the way.
That would have a run time of O(n) though. I think we can do better.
We know that the array is already sorted, so we could use binary search here. That would allow us to search in O(log n) time, which would be more efficient than linear search, unless the input array was very small at least.
Should I assume the input is likely to be large?
This kind of discussion is important because it allows you to validate your plan and make sure that it is actually what the interviewer is looking for. Listen carefully for hints, as they might try to point you in a different direction, or highlight something you haven't considered.
An example from Google
This mock interview does a good job of demonstrating the how the discussion during a technical interview should go. They aren't as thorough in some of the other areas that I'm describing, though.
Once the interviewer agrees that your solution sounds good, write it in pseudocode. This has two benefits: you can focus on getting the logic right without worrying about syntax, and then you can use it as a reference as you write your actual code (which should be super easy as it's basically just copying by that point).
A pseudocode example of the above problem would be:
function binary_search(A, n, T) is
L := 0
R := n โ 1
while L โค R do
m := floor((L + R) / 2)
if A[m] < T then
L := m + 1
else if A[m] > T then
R := m โ 1
else:
return m
return unsuccessful
5. Write the actual code
It's taken 5 steps to get here, but you're finally ready to code your actual solution.
This should be straightforward by this point. You have test cases, you have a plan, you have a reference to copy, and you know exactly what you're going to doโso it really should be a case of now just translating all of this into real code.
This means you can take the time to do all the things people don't usually do when they write code in interviews: naming things well, making your code readable, making sure syntax is correct, adding comments where necessary...
Many interviewers won't be expecting these things, so while not strictly necessary, this kind of care and attention to detail is a big plus.
6. Step through line by line with test cases
You might think you're done once your code is done, but this is another place people slip up. It's easy to breathe a sigh of relief and declare your solution ready, but you need to go back and make sure it's actually doing what you think it is.
If you wrote real unit tests, this part becomes a lot easier. Run them to prove that your code has satisfied all the requirements.
If you don't have actual tests, you should step through the code, line by line, with each inputs/output combination from your test cases. Describe what would happen at each line, and confirm that you didn't make any mistakes.
Don't just skim over this part; it's really easy to just assume your code works and miss bugs. The point of this is to identify mistakes. It's fine if you did make a mistake, as long as you catch it in this step and correct it.
Your interviewer will also be looking out for bugs, so communicate out loud as you step through your code, and listen carefully to any feedback (they might spot something you didn't).
7. Optimize and discuss additional considerations
If you followed all the other steps, you've hopefully got a well-planned, working solution, and have likely already passed the interview. But there could still be some extra optimizations you could talk through, for bonus points.
You probably won't write more code at this stage, but use the time to consider if there's anything you could do to make your solution better. Could you improve the running time any further? Are there any cases this solution wouldn't work for (and how could you adapt it to work for those too)? Would you do anything differently if you had more time?
Think outside the parameters the interviewer gave you earlier. This shows you can engage with problems in the wider context, beyond just the bare minimum requirements.
Final thoughts
I know this process might sound like a lot, but it doesn't take too long to go through these steps in a real interview.
Of course, you do still need to study the fundamentals and practice answering questions beforehand. But if you can use a structured approach and communicate like I've described in the actual interview, you'll stand a much better chance.
It's certainly paid off in all the interviews I've used it inโI hope it can do the same for you!